Pre-prep:
- Installed Windows 8 Release Preview
- Installed VS 2012
- Did some exploration of the Bing Maps sdk for metro (blogged about it here: Using Bing Maps in a Metro App)
Idea: Port my OptiRoute Windows Phone 7 app to Windows 8
8:30 am : Check in. Setup my area, pickup coffee!
9:00 am : Quick sketch of how I plan on migrating OptiRoute to Windows 8. Setup a default VS project based on the “Grid App”
9:15 am: uh! oh! – VS 2012 preview does not open my Windows Phone 7 projects:
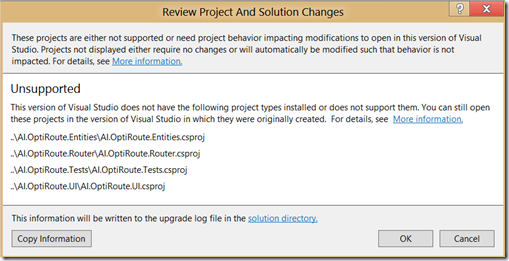
Need to install VS 2010 if I need to open it – screw – I just need the code – NotePad++ to the rescue.
9:30 am: Came up with basic design on paper. Watching Jerry Nixon’s demo.
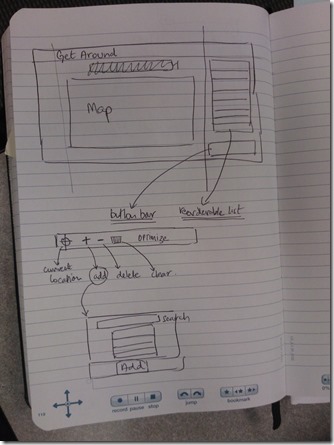
10:00 am: Jerry Nixon’s demo is still going on. First issue encountered. Added reference to the Bing Maps Geocode Service. Looks like in WinRT there is no “GeocodeCompleted” event to which I can add my own handler. Hmmm, looks like I need to use “Await Async” model.
This Windows Phone 7 code changes: public class GeoCoder { public void GeoCode(string query, Action<List<Coordinate>> action) { GeocodeServiceClient client = new GeocodeServiceClient(); client.GeocodeCompleted += new EventHandler<GeocodeCompletedEventArgs>(client_GeocodeCompleted); GeocodeRequest request = new GeocodeRequest(); request.Credentials = new Credentials(); request.Credentials.ApplicationId = "Your Bing Maps Service Key Here"; request.Query = query; client.GeocodeAsync(request, action); } void client_GeocodeCompleted(object sender, GeocodeCompletedEventArgs e) { if (!e.Cancelled && e.Error == null) { Action<List<Coordinate>> action = e.UserState as Action<List<Coordinate>>; List<Coordinate> coordinates = new List<Coordinate>(); foreach (GeocodeResult geocodeResult in e.Result.Results) { foreach (GeocodeLocation geocodeLocation in geocodeResult.Locations) { coordinates.Add(new Coordinate(geocodeLocation.Latitude, geocodeLocation.Longitude)); } } action(coordinates); } } } |
Here is what the code looks like in WinRT:
async public Task<List<Coordinate>> GeoCode(string query) { GeocodeServiceClient client = new GeocodeServiceClient(GeocodeServiceClient.EndpointConfiguration.BasicHttpBinding_IGeocodeService); GeocodeRequest request = new GeocodeRequest(); request.Credentials = new Credentials(); request.Credentials.ApplicationId = "Bing Maps Service Key Here"; request.Query = query; var geoCodeResponse = await client.GeocodeAsync(request); List<Coordinate> coordinates = new List<Coordinate>(); if (geoCodeResponse != null) { foreach (GeocodeResult geocodeResult in geoCodeResponse.Results) { foreach (GeocodeLocation geocodeLocation in geocodeResult.Locations) { coordinates.Add(new Coordinate(geocodeLocation.Latitude, geocodeLocation.Longitude)); } } } return coordinates; } |
Wow! that code is so much more nicer looking! Me like this new Asynchronous programming model.
10:30 am: Jerry is still demoing his rad WinRT dev skills. Using geo-location, Netflix OData feeds to make an app.
10:31 am: For the last 15 minutes was fighting with a unit test project to get an Async test to run. It would not run!!
Took out the async keyword and it ran! weird!
Modified the unit test to not use the “await” keyword, which meant I could take out the “async” qualifier on the method.
Test is passing – woo! hoo! getting geo-coded results for my query!
[TestMethod] public void TestGeoCoder() { var geoCoder = new Bing.GeoCoder.GeoCoder(); var asyncReturn = geoCoder.GeoCode("1 Microsoft Way"); List<Coordinate> coordinates = asyncReturn.Result; Assert.IsNotNull(coordinates); Assert.IsTrue(coordinates.Count > 0); }
|
Also, needed to update the manifest of the test project to allow for internet connectivity for the tests to pass!
10:45 am: Jerry is finally done with his demo! He created a complete app in about 1 hour.
10:47 am: Looks like I cant call “Array.Sort(array,array)” (where the first array are the values and 2nd are the keys) method in a Metro app. Why would they have dropped this method?!!! WTF!
Need to implement my own code for this! (Note to self – see if I can get rid of the need for this sort completely).
For now, using a List of KeyValuePairs, ordering by the key and then using Linq to select the sorted set of keys
11:00 am: Looking good. All tests passing!
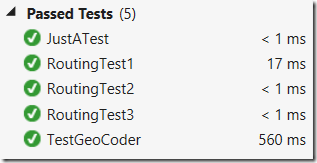
11:05 am: Migrated most of my base routing algorithm code. Now on to the UI
11:20 am: Working on the UI.
One needs to remember to set the active configuration platform to x86 for Bing Maps to work in the designer (x64 is not supported by the designer)
12:10 pm: Its lunch time. Still working on the UI
1:30 pm: Called in Jerry to help me with setting up a wrap panel. This is why these events are so cool. You get resources to help you through some of your road blocks. Thanks Jerry!
3:20 pm: Encountered a bunch of road blocks that I had to work through:
The biggest of which was that the map control has completely been changed in how things are done with it in the Metro SDK. I am still trying to figure out a lot of the stuff that I need to make the app ready for prime time (eg: Pushpin’s cant have color, line widths arent working correctly, unable to get the view rectangle, etc).
But here is where I am:
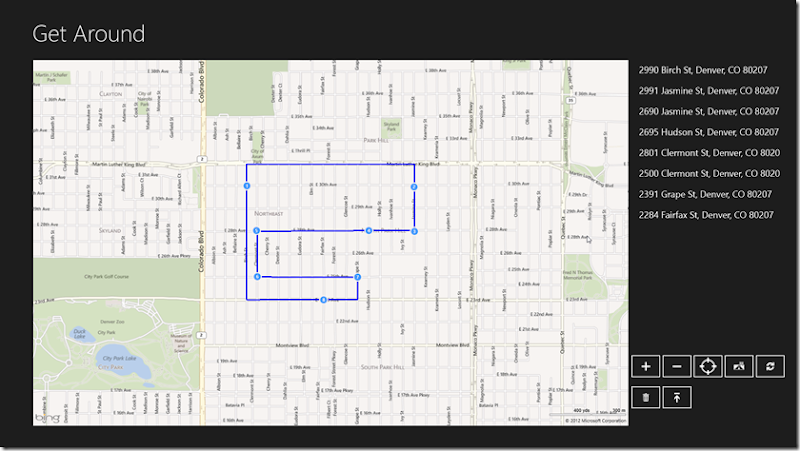
6:30pm: Things are coming along real nice and I am feeling nice about my app. Lots of unfinished features, but here is what I have gotten done now:
1. Loading a sample route.
2. Optimizing the sample route
3. Fly out menu to change map type (road, aerial, birds eye)
4. Sharing via email – share the route.
5. Searching for locations
7:15 pm: And we are done. Now the presentations start – Best of luck to me!
7:30 pm: Crap my application package is not working on the demo computer. Its only my package that’s having an error during installation. Jerry thinks its because I am using Bing maps and some dependency is not resolving. Thought I could demo it using my laptop. Nope cant do that – my laptop doesn’t have a VGA port (note to self, buy a HDMI to VGA convertor and keep in bag)
8:00 pm: I have been reschedule to come on at the end. Jerry forgot! He is asking everyone to vote! WAIT…. Jerry has an idea, use his laptop. It works. App is installed and I am going to demo it now!
8:03 pm: 3 minutes fly by during a presentation. Hit upon all the major features. Crossing my fingers.
8:30 pm: 17 apps were presented. 3 apps won the grand prize (Slate + Gift Card). My app (Get Around!) was one of them. I am stoked!
Parting thoughts:
The dev intro event to Win8 on Friday and the hackathon on saturday were well organized. Microsoft had every needed resource on hand. Xaml experts, Javascript experts, sys-admins to help installing Win8, great food and awesome give-aways and prizes. All we had to do was come with our laptops. Jerry Nixon did a great job running the whole show.
Here is what my completed app looked like:
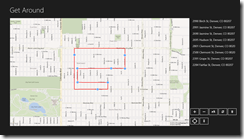 | 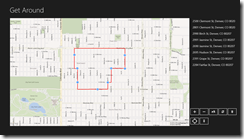 | 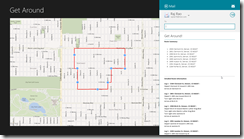 | |
Initial un-optimized route | Optimized route | Sharing route via email | |
Links:
Jerry Nixon: http://blog.jerrynixon.com/
Win 8 resources used during the dev event: http://blog.jerrynixon.com/2012/08/windows-8-resources-links-to-get.html
Joe Shirey: http://www.joeshirey.com/
Michael Palermo: http://palermo4.com/
Harold Wong: http://blogs.technet.com/b/haroldwong/ (has great post on installing Win8 using VHD. Also has posted VHDs that you can use to setup Win8 on your machine)